一、从 Gradle 和 AS 开始
When Google introduced Gradle and Android Studio, they had some goals in mind. They wanted to make it easier to reuse code, create build variants, and configure and customize the build process. On top of that, they wanted good IDE integration, but without making the build system dependent on the IDE. Running Gradle from the command line or on a continuous integration server will always yield the same results as running a build from Android Studio.
We will refer to Android Studio occasionally throughout the book, because it often provides a simpler way of setting up projects, dealing with changes, and so on. If you do not have Android Studio installed yet, you can download it from the Android developer website ( http://developer.android.com/sdk/index.html ).
In this chapter, we will cover the following topics:
- Getting to know Android Studio
- Understanding Gradle basics
- Creating a new project
- Getting started with the Gradle wrapper
- Migrating from Eclipse
当Google推出Gradle和Android Studio时,他们有一些目标。他们希望能够更轻松地重用代码,创建构建变量,以及配置和定制构建过程。最重要的是,他们希望有良好的IDE集成,但是不要使构建系统依赖于IDE。通过命令行或持续集成服务器运行Gradle将始终产生与通过Android Studio运行构建相同的结果。
我们将在本书中偶尔提及Android Studio,因为它经常提供一个更简单的方法来设置项目,处理更改等等。 如果您还没有安装Android Studio,可以从Android开发者网站下载。
在本章中,我们将介绍以下主题:
- 开始了解Android Studio
- 了解Gradle基础
- 创建一个新的项目
- 开始使用Gradle wrapper
- 从Eclipse迁移
理解基本的Gradle *
Project和tasks
在gradle中有两大重要的概念,分别是project和tasks。
每一个构建都由至少一个project组成,每个project都至少包含一个tasks。
每一个build.gradle文件代表着一个project,tasks在build.gradle中定义。
当初始化构建进程时,gradle会基于build文件,组合所有的project和tasks。
一个tasks包含了一系列的动作,它们会按照顺序执行。
一个动作就是一段被执行的代码,它们很像Java中的方法。
build生命周期
一次构建将会经历下列三个阶段:
- 初始化阶段:project实例在这儿创建,如果有多个模块,即有多个build.gradle文件,多个project将会被创建。
- 配置阶段:在该阶段,build.gradle脚本将会执行,为每个project创建和配置所有的tasks。
- 执行阶段:这一阶段,gradle会决定哪一个tasks会被执行。哪一个tasks会被执行完全依赖于,开始构建时传入的参数,和当前所在的文件夹位置等。
build配置文件
In the repositories block, the JCenter repository is configured as a source of dependencies for the build script. JCenter is a preconfigured Maven repository and requires no extra setup; Gradle has you covered. There are several repositories available straight from Gradle and it is easy to add your own, either local or remote.
The build script block also defines a dependency on Android build tools as a classpath Maven artifact. This is where the Android plugin comes from. The Android plugin provides everything needed to build and test applications. Every Android project needs to apply the Android plugin using this line:
apply plugin: 'com.android.application'
Plugins are used to extend the capabilities of a Gradle build script. Applying a plugin to a project makes it possible for the build script to define properties and use tasks that are defined in the plugin.
If you are building a library, you need to apply 'com.android. library' instead. You cannot use both in the same module because that would result in a build error. A module can be either an Android application or an Android library, not both.
When using the Android plugin, Android-specific conventions can be configured and tasks only applicable to Android will be generated. The Android block in the following snippet is defined by the plugin and can be configured per project:
android { compileSdkVersion 22 buildToolsVersion "22.0.1" }
This is where the Android-specific part of the build is configured. The Android plugin provides a DSL tailored to Android's needs. The only required properties are the compilation target and the build tools. The compilation target, specified by compileSdkVersion , is the SDK version that should be used to compile the app. It is good practice to use the latest Android API version as the compilation target.
There are plenty of customizable properties in the build.gradle file. We will discuss the most important properties in Chapter 2, Basic Build Customization, and more possibilities throughout the rest of the book.
- buildscript {
- repositories {
- jcenter() //存储库
- }
- dependencies {
- classpath 'com.android.tools.build:gradle:1.2.3'
- }
- }
buildscript {
repositories {
jcenter() //存储库
}
dependencies {
classpath 'com.android.tools.build:gradle:1.2.3'
}
}
这就是对实际构建所做的配置。
在存储库块中,JCenter存储库被配置为构建脚本的依赖关系的来源。 JCenter是一个预先配置的Maven仓库,不需要额外的设置,Gradle中有你的覆盖。从Gradle中可以直接找到几个存储库,很容易添加你自己的或本地的或远程的。
构建脚本块还将Android构建工具的依赖性定义为类路径Maven构件。这就是Android plugin的来源之处。Android插件提供了构建和测试应用程序所需的一切。每个Android项目都需要使用这一行来应用Android插件:
apply plugin: 'com.android.application'
apply plugin: 'com.android.application'
插件用于扩展Gradle构建脚本的功能。在一个项目中使用插件,这样该项目的构建脚本就可以定义该插件定义好的属性和使用它的tasks。
注意:当你在开发一个依赖库时,你应该使用:
apply plugin: 'com.android.library'
apply plugin: 'com.android.library'
你不能在同一个模块中使用两者,这会导致构建错误。模块可以是Android应用程序或Android库,而不能两者都是。
使用Android插件时,可以配置特定于Android的约定,并仅生成适用于Android的task。以下代码段中的Android代码块由插件定义,可以为每个项目配置:
- android {
- compileSdkVersion 22
- buildToolsVersion "22.0.1"
- }
android {
compileSdkVersion 22
buildToolsVersion "22.0.1"
}
这是构建Android特定部分的地方。Android插件提供了一个适合Android需求的DSL。唯一需要的属性是编译目标和构建工具。由compileSdkVersion指定的编译目标是用于编译应用程序的SDK版本。使用最新的Android API版本作为编译目标是一个很好的做法。
build.gradle文件中有很多可定制的属性。我们将在第二章“基本构建定制”中讨论最重要的属性,以及通过本书其余部分讨论更多可能性。
项目结构
- MyApp
- ├── build.gradle
- ├── settings.gradle
- └── app
- ├── build.gradle
- ├── build //The output of the build process
- ├── libs //These are external libraries (.jar or .aar)
- └── src
- └── main
- ├── java //The source code for the app
- │ └── com.package.myapp
- └── res //These are app-related resources (drawables, layouts, strings, and so on)
- ├── drawable
- ├── layout
- └── etc.
MyApp
├── build.gradle
├── settings.gradle
└── app
├── build.gradle
├── build //The output of the build process
├── libs //These are external libraries (.jar or .aar)
└── src
└── main
├── java //The source code for the app
│ └── com.package.myapp
└── res //These are app-related resources (drawables, layouts, strings, and so on)
├── drawable
├── layout
└── etc.
Gradle使用了一个叫做source set的概念,Gradle官方文档解释说:一个source set就是一系列资源文件,它们被一起编译和执行。对于Android项目,main就是一个包含了应用程序默认版本所有源代码和资源的source set。当你开始为你的Android应用程序编写测试时,你将把测试的源代码放在一个名为androidTest的独立源代码集中,它只包含测试。
Gradle Wrapper入门
获取Gradle Wrapper
【Getting the Gradle Wrapper】
For your convenience, every new Android project includes the Gradle Wrapper, so when you create a new project, you do not have to do anything at all to get the necessary files. It is, of course, possible to install Gradle manually on your computer and use it for your project, but the Gradle Wrapper can do the same things and guarantee that the correct version of Gradle is used. There is no good reason not to use the wrapper when working with Gradle outside of Android Studio.
You can check if the Gradle Wrapper is present in your project by navigating to the project folder and running ./gradlew –v from the terminal or gradlew.bat –v from Command Prompt. Running this command displays the version of Gradle and some extra information about your setup. If you are converting an Eclipse project, the wrapper will not be present by default. In this case, it is possible to generate it using Gradle, but you will need to install Gradle first to get the wrapper.
After you have downloaded and installed Gradle and added it to your PATH , create a build.gradle file containing these three lines:
task wrapper(type: Wrapper) { gradleVersion = '2.4' }
After that, run gradle wrapper to generate the wrapper files.
In recent versions of Gradle, you can also run the wrapper task without modifying the build.gradle file, because it is included as a task by default. In that case, you can specify the version with the --gradle-version parameter, like this:
$ gradle wrapper --gradle-version 2.4
If you do not specify a version number, the wrapper is configured to use the Gradle version that the task is executed with.
These are all the files generated by the wrapper task:...
The gradle-wrapper.properties file is the one that contains the configuration and determines what version of Gradle is used:...
You can change the distribution URL if you want to use a customized Gradle distribution internally. This also means that any app or library that you use could have a different URL for Gradle, so be sure to check whether you can trust the properties before you run the wrapper.
Android Studio is kind enough to display a notification when the Gradle version used in a project is not up to date and will suggest automatically updating it for you. Basically, Android Studio changes the configuration in the gradle-wrapper. properties file and triggers a build, so that the latest version gets downloaded.
PS:Android Studio uses the information in the properties to determine which version of Gradle to use, and it runs the wrapper from the Gradle Wrapper directory inside your project. However, it does not make use of the shell or bash scripts, so you should not customize those.
为了方便起见,每个新的Android项目都包含了Gradle Wrapper,所以当你创建一个新的项目时,你不需要做任何事情来获取必要的文件。当然,可以在您的计算机上手动安装Gradle,并将其用于您的项目,但Gradle Wrapper可以做同样的事情,并保证使用正确版本的Gradle。在Android Studio之外使用Gradle时,没有理由不使用Gradle Wrapper。
您可以通过导航到项目文件夹并从终端或命令行运行【gradlew -v】或【gradlew.bat -v】来检查项目中是否存在Gradle Wrapper。运行此命令将显示Gradle的版本以及有关您设置的一些额外信息。


如果你正在转换一个Eclipse项目,默认情况下,wrapper将不会出现。 在这种情况下,可以使用Gradle生成它,但是您需要首先安装Gradle来获取wrapper。
在您下载并安装Gradle并将其添加到PATH后,创建一个包含以下三行的 build.gradle 文件(白注:在根目录下的 build.gradle 文件中添加以下代码即可):
- task wrapper(type: Wrapper) {
- gradleVersion = '2.4'
- }
x
task wrapper(type: Wrapper) {
gradleVersion = '2.4'
}
之后,运行Gradle Wrapper来生成Wrapper文件(白注:添加上述代码后同步一下即可生成完整的 gradle 目录)。
在最近的Gradle版本中,你也可以在不修改 build.gradle 文件的情况下运行 wrapper task,因为默认情况下它被包含为一个task。在这种情况下,您可以使用【--gradle-version】参数指定版本,如下所示:
gradle wrapper --gradle-version 2.4
gradle wrapper --gradle-version 2.4
如果不指定版本号,则将wrapper配置为使用执行task的Gradle版本。
下列是由wrapper task生成的所有文件:
- myapp/
- ├── gradlew //针对Linux和Mac系统的shell脚本文件, A shell script on Linux and Mac OS X
- ├── gradlew.bat //针对windows系统的bat文件,A batch file on Microsoft Windows
- └── gradle/wrapper/
- ├── gradle-wrapper.jar //A JAR file that is used by the batch file and shell script
- └── gradle-wrapper.properties //配置文件,A properties file,用于确定使用哪个版本的Gradle
x
myapp/
├── gradlew //针对Linux和Mac系统的shell脚本文件, A shell script on Linux and Mac OS X
├── gradlew.bat //针对windows系统的bat文件,A batch file on Microsoft Windows
└── gradle/wrapper/
├── gradle-wrapper.jar //A JAR file that is used by the batch file and shell script
└── gradle-wrapper.properties //配置文件,A properties file,用于确定使用哪个版本的Gradle
【gradle-wrapper.properties】文件是一个包含配置并确定使用哪个版本的Gradle的文件:
- #Sat May 30 17:41:49 CEST 2015
- distributionBase=GRADLE_USER_HOME
- distributionPath=wrapper/dists
- zipStoreBase=GRADLE_USER_HOME
- zipStorePath=wrapper/dists
- distributionUrl=https\://services.gradle.org/distributions/gradle-2.4-all.zip
#Sat May 30 17:41:49 CEST 2015
distributionBase=GRADLE_USER_HOME
distributionPath=wrapper/dists
zipStoreBase=GRADLE_USER_HOME
zipStorePath=wrapper/dists
distributionUrl=https\://services.gradle.org/distributions/gradle-2.4-all.zip
如果您想在内部使用定制的Gradle,则可以更改URL(可以使用本地路径)。这也意味着您使用的任何应用程序或库都可能具有不同的Gradle URL,因此请务必在运行wrapper之前检查是否可以信任这些属性。
如果项目中使用的Gradle版本不是最新的,Android Studio会提供足够的通知,并且会建议您自动更新。基本上,Android Studio更改gradle-wrapper.properties中的配置并触发构建,以便下载最新版本。
注意:Android Studio使用properties中的信息来确定使用哪个版本的Gradle,并运行你工程中Gradle Wrapper目录的wrapper。但是,它并没有使用shell或bash脚本,所以你不应该自定义这些。
运行基本build任务
- check:runs all the checks, this usually means running tests on a connected device or emulator
- build:triggers both assemble and check
- clean:cleans the output of the project
gradlew tasks
gradlew tasks
这将打印出所有可用task列表(白注:第一次使用此命令会下载一些东西,大概要六到八分钟)。如果添加--all参数,则可以获得有关每个task的依赖性的更详细概述。
白注:这些列表和AS中Gradle面板中的一致。
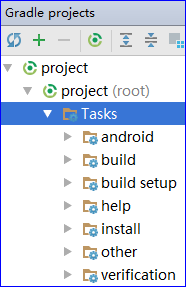
- > Task :tasks
-
- ------------------------------------------------------------
- All tasks runnable from root project
- ------------------------------------------------------------
-
- Android tasks
- -------------
- androidDependencies - Displays the Android dependencies of the project.
- signingReport - Displays the signing info for each variant.
- sourceSets - Prints out all the source sets defined in this project.
-
- Build tasks
- -----------
- assemble - Assembles all variants of all applications and secondary packages.
- assembleAndroidTest - Assembles all the Test applications.
- assembleDebug - Assembles all Debug builds.
- assembleRelease - Assembles all Release builds.
- build - Assembles and tests this project.
- buildDependents - Assembles and tests this project and all projects that depend on it.
- buildNeeded - Assembles and tests this project and all projects it depends on.
- clean - Deletes the build directory.
- cleanBuildCache - Deletes the build cache directory.
- compileDebugAndroidTestSources
- compileDebugSources
- compileDebugUnitTestSources
- compileReleaseSources
- compileReleaseUnitTestSources
- mockableAndroidJar - Creates a version of android.jar that's suitable for unit tests.
- Build Setup tasks
- -----------------
- init - Initializes a new Gradle build.
- Help tasks
- ----------
- buildEnvironment - Displays all buildscript dependencies declared in root project 'project'.
- components - Displays the components produced by root project 'project'. [incubating]
- dependencies - Displays all dependencies declared in root project 'project'.
- dependencyInsight - Displays the insight into a specific dependency in root project 'project'.
- dependentComponents - Displays the dependent components of components in root project 'project'. [incubating]
- help - Displays a help message.
- model - Displays the configuration model of root project 'project'. [incubating]
- projects - Displays the sub-projects of root project 'project'.
- properties - Displays the properties of root project 'project'.
- tasks - Displays the tasks runnable from root project 'project' (some of the displayed tasks may belong to subprojects).
- Install tasks
- -------------
- installDebug - Installs the Debug build.
- installDebugAndroidTest - Installs the android (on device) tests for the Debug build.
- uninstallAll - Uninstall all applications.
- uninstallDebug - Uninstalls the Debug build.
- uninstallDebugAndroidTest - Uninstalls the android (on device) tests for the Debug build.
- uninstallRelease - Uninstalls the Release build.
- Verification tasks
- ------------------
- check - Runs all checks.
- connectedAndroidTest - Installs and runs instrumentation tests for all flavors on connected devices.
- connectedCheck - Runs all device checks on currently connected devices.
- connectedDebugAndroidTest - Installs and runs the tests for debug on connected devices.
- deviceAndroidTest - Installs and runs instrumentation tests using all Device Providers.
- deviceCheck - Runs all device checks using Device Providers and Test Servers.
- lint - Runs lint on all variants.
- lintDebug - Runs lint on the Debug build.
- lintRelease - Runs lint on the Release build.
- lintVitalRelease - Runs lint on just the fatal issues in the release build.
- test - Run unit tests for all variants.
- testDebugUnitTest - Run unit tests for the debug build.
- testReleaseUnitTest - Run unit tests for the release build.
- To see all tasks and more detail, run gradlew tasks --all
- To see more detail about a task, run gradlew help --task <task>
x
> Task :tasks
------------------------------------------------------------
All tasks runnable from root project
------------------------------------------------------------
Android tasks
-------------
androidDependencies - Displays the Android dependencies of the project.
signingReport - Displays the signing info for each variant.
sourceSets - Prints out all the source sets defined in this project.
Build tasks
-----------
assemble - Assembles all variants of all applications and secondary packages.
assembleAndroidTest - Assembles all the Test applications.
assembleDebug - Assembles all Debug builds.
assembleRelease - Assembles all Release builds.
build - Assembles and tests this project.
buildDependents - Assembles and tests this project and all projects that depend on it.
buildNeeded - Assembles and tests this project and all projects it depends on.
clean - Deletes the build directory.
cleanBuildCache - Deletes the build cache directory.
compileDebugAndroidTestSources
compileDebugSources
compileDebugUnitTestSources
compileReleaseSources
compileReleaseUnitTestSources
mockableAndroidJar - Creates a version of android.jar that's suitable for unit tests.
Build Setup tasks
-----------------
init - Initializes a new Gradle build.
Help tasks
----------
buildEnvironment - Displays all buildscript dependencies declared in root project 'project'.
components - Displays the components produced by root project 'project'. [incubating]
dependencies - Displays all dependencies declared in root project 'project'.
dependencyInsight - Displays the insight into a specific dependency in root project 'project'.
dependentComponents - Displays the dependent components of components in root project 'project'. [incubating]
help - Displays a help message.
model - Displays the configuration model of root project 'project'. [incubating]
projects - Displays the sub-projects of root project 'project'.
properties - Displays the properties of root project 'project'.
tasks - Displays the tasks runnable from root project 'project' (some of the displayed tasks may belong to subprojects).
Install tasks
-------------
installDebug - Installs the Debug build.
installDebugAndroidTest - Installs the android (on device) tests for the Debug build.
uninstallAll - Uninstall all applications.
uninstallDebug - Uninstalls the Debug build.
uninstallDebugAndroidTest - Uninstalls the android (on device) tests for the Debug build.
uninstallRelease - Uninstalls the Release build.
Verification tasks
------------------
check - Runs all checks.
connectedAndroidTest - Installs and runs instrumentation tests for all flavors on connected devices.
connectedCheck - Runs all device checks on currently connected devices.
connectedDebugAndroidTest - Installs and runs the tests for debug on connected devices.
deviceAndroidTest - Installs and runs instrumentation tests using all Device Providers.
deviceCheck - Runs all device checks using Device Providers and Test Servers.
lint - Runs lint on all variants.
lintDebug - Runs lint on the Debug build.
lintRelease - Runs lint on the Release build.
lintVitalRelease - Runs lint on just the fatal issues in the release build.
test - Run unit tests for all variants.
testDebugUnitTest - Run unit tests for the debug build.
testReleaseUnitTest - Run unit tests for the release build.
To see all tasks and more detail, run gradlew tasks --all
To see more detail about a task, run gradlew help --task <task>
注意:在Microsoft Windows上,您需要运行gradlew.bat,在Linux和Mac OS X上,完整的命令是./gradlew。为了简洁起见,我们在整本书中只是写作gradlew 。
要在开发过程中构建项目,请使用调试配置运行组合的task(白注:第一次使用此命令也会下载一些东西):
gradlew assembleDebug
gradlew assembleDebug
此task将使用该应用程序的调试版本创建一个APK(白注:这和在Gradle面板中双击 build/assembleDebug 的效果一致)。默认情况下,Gradle的Android插件将APK保存在 MyApp/app/build/outputs/apk 目录中。
注意:使用缩写的task名称是为了避免在终端中输入大量的内容,Gradle还提供骆驼风格缩写的task名称作为快捷键。例如,可以通过从命令行界面运行gradlew assDeb来执行assembleDebug,甚至可以是aD。但这里有一个警告,仅仅当骆驼风格的缩写是唯一的情况下它才会起作用。一旦另一个任务具有相同的缩写,这个技巧就不再适用于这些task。
除了assemble外,还有另外三个基本的任务:
- check:运行所有检查,这通常意味着在连接的设备或模拟器上运行测试
- build:触发assemble和check命令
- clean:清理项目的输出
我们将在第2章基本构建定制中详细讨论这些任务。
总结
- 1.1 Android Studio
- 1.1.1 保持最新 Staying up to date
- 1.3 创建新项目 Creating a new project
- 1.5 迁移出Eclipse Migrating from Eclipse
- 1.5.1 使用导入向导 Using the import wizard
- 1.5.2 手动迁移 Migrating manually
- 保持旧的项目结构 Keeping the old project structure
- 转换到新的项目结构 Converting to the new project structure
- 迁移库 Migrating libraries
1.1 Android Studio
1.1.1 保持最新 Staying up to date
1.3 创建新项目 Creating a new project
1.5 迁移出Eclipse Migrating from Eclipse
1.5.1 使用导入向导 Using the import wizard
1.5.2 手动迁移 Migrating manually
保持旧的项目结构 Keeping the old project structure
转换到新的项目结构 Converting to the new project structure
迁移库 Migrating libraries